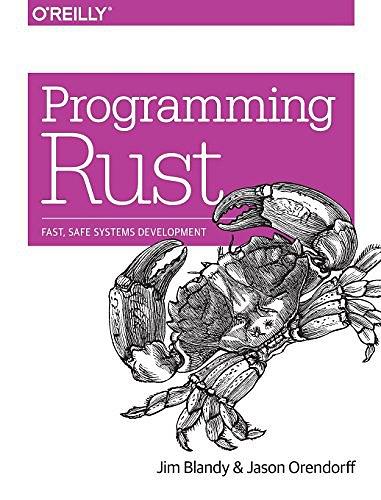
书名:Programming RustFast,SafeSystemsDevelopment
作者:JimBlandy/JasonOrendorff
译者:
ISBN:9781491927281
出版社:O'ReillyMedia
出版时间:2016-8-25
格式:epub/mobi/azw3/pdf
页数:400
豆瓣评分: 8.8
书籍简介:
This practical book introduces systems programmers to Rust, the new and cutting-edge language that’s still in the experimental/lab stage. You’ll learn how Rust offers the rare and valuable combination of statically verified memory safety and low-level control—imagine C++, but without dangling pointers, null pointer dereferences, leaks, or buffer overruns. Author Jim Blandy—the maintainer of GNU Emacs and GNU Guile—demonstrates how Rust has the potential to be the first usable programming language that brings the benefits of an expressive modern type system to systems programming. Rust’s rules for borrowing, mutability, ownership, and moves versus copies will be unfamiliar to most systems programmers, but they’re key to Rust’s unique advantages. This book presents Rust’s rules clearly and economically; elaborates on their consequences; and shows you how to express the programs you want to write in terms that Rust can prove are free of a broad class of common errors.
作者简介:
Jim Blandy has been programming since 1981, and writing Free software since 1990. He has been the maintainer of GNU Emacs and GNU Guile, and a maintainer of GDB, the GNU Debugger. He is one of the original designers of the Subversion version control system. Jim now works on Firefox’s web developer tools for Mozilla.
书友短评:
@ paranoid.emacs 我觉得最好的系统语言还是modern c++. @ 10/10=1 读到了对Rust更详细的认识。 @ HariSeldon 比官方好 @ 我就改个名字 大略看了一遍,讲的比较细,不适合入门。参考书。 @ xiaq 讲 trait 的地方能让人感觉到 rust 是一个还没设计完的语言。 @ モナドハンター rust是个好语言,希望能早日取代c吧 @ TING 认真严肃的定期书友是学习的一大助力。。。 @ Yuumu Konpaku out of date @ 我就改个名字 大略看了一遍,讲的比较细,不适合入门。参考书。 @ chai2010 灵魂讲透了
Preface
1. Why Rust?
Type Safety
2. A Tour of Rust
Downloading and Installing Rust
A Simple Function
Writing and Running Unit Tests
Handling Command-Line Arguments
A Simple Web Server
Concurrency
3. Basic Types
Machine Types
Tuples
Pointer Types
Arrays, Vectors, and Slices
String Types
Beyond the Basics
4. Ownership
Ownership
Moves
Copy Types: The Exception to Moves
Rc and Arc: Shared Ownership
5. References
References as Values
Reference Safety
Sharing Versus Mutation
Taking Arms Against a Sea of Objects
6. Expressions
An Expression Language
Blocks and Semicolons
Declarations
if and match
Loops
return Expressions
Why Rust Has loop
Function and Method Calls
Fields and Elements
Reference Operators
Arithmetic, Bitwise, Comparison, and Logical Operators
Assignment
Type Casts
Closures
Precedence and Associativity
Onward
7. Error Handling
Panic
Result
8. Crates and Modules
Crates
Modules
Turning a Program into a Library
The src/bin Directory
Attributes
Tests and Documentation
Specifying Dependencies
Publishing Crates to crates.io
Workspaces
More Nice Things
9. Structs
Named-Field Structs
Tuple-Like Structs
Unit-Like Structs
Struct Layout
Defining Methods with impl
Generic Structs
Structs with Lifetime Parameters
Deriving Common Traits for Struct Types
Interior Mutability
10. Enums and Patterns
Enums
Patterns
The Big Picture
11. Traits and Generics
Using Traits
Defining and Implementing Traits
Fully Qualified Method Calls
Traits That Define Relationships Between Types
Reverse-Engineering Bounds
Conclusion
12. Operator Overloading
Arithmetic and Bitwise Operators
Equality Tests
Ordered Comparisons
Index and IndexMut
Other Operators
13. Utility Traits
Drop
Sized
Clone
Copy
Deref and DerefMut
Default
AsRef and AsMut
Borrow and BorrowMut
From and Into
ToOwned
Borrow and ToOwned at Work: The Humble Cow
14. Closures
Capturing Variables
Function and Closure Types
Closure Performance
Closures and Safety
Callbacks
Using Closures Effectively
15. Iterators
The Iterator and IntoIterator Traits
Creating Iterators
Iterator Adapters
Consuming Iterators
Implementing Your Own Iterators
16. Collections
Overview
Vec<T>
VecDeque<T>
LinkedList<T>
BinaryHeap<T>
HashMap<K, V> and BTreeMap<K, V>
HashSet<T> and BTreeSet<T>
Hashing
Beyond the Standard Collections
17. Strings and Text
Some Unicode Background
Characters (char)
String and str
Formatting Values
Regular Expressions
Normalization
18. Input and Output
Readers and Writers
Files and Directories
Networking
19. Concurrency
Fork-Join Parallelism
Channels
Shared Mutable State
What Hacking Concurrent Code in Rust Is Like
20. Macros
Macro Basics
Built-In Macros
Debugging Macros
The json! Macro
Avoiding Syntax Errors During Matching
Beyond macro_rules!
21. Unsafe Code
Unsafe from What?
Unsafe Blocks
Unsafe Functions
Unsafe Block or Unsafe Function?
Undefined Behavior
Unsafe Traits
Raw Pointers
Foreign Functions: Calling C and C++ from Rust
Conclusion
Index
· · · · · ·
添加微信公众号:好书天下获取
评论前必须登录!
注册